Step-by-Step Guide: Creating a Dynamic Form with KuFlow
Published 11-09-2023 by Hector Tessari - KuFlow Team
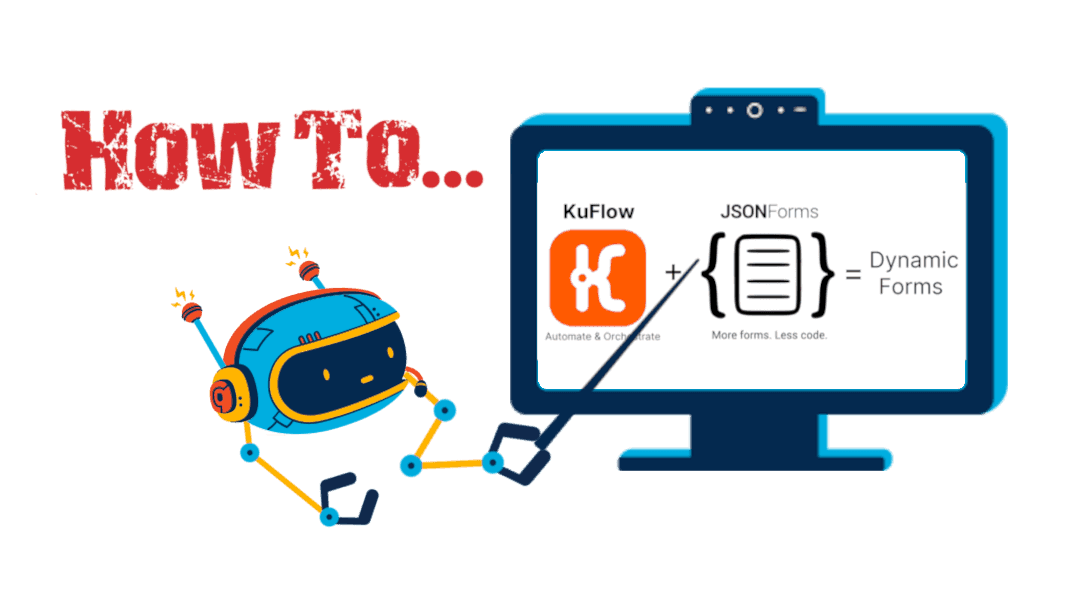
Credits: Created by Hector Tessari
Creating dynamic forms doesn't have to be complicated. With KuFlow's integration of JSON Forms, you can easily build intuitive and user-friendly forms tailored to your needs. In this guide, we'll take you through the process step by step, starting from the simplest form and gradually building up to a more complex example.
Step 1: Creating a Basic Form
Let's start with a basic form that captures personal information such as name, surname, age, birthdate, and nationality. Here's how the Schema and UI Schema would look:
// Schema
{
"title": "A form",
"description": "A simple form example",
"type": "object",
"definitions": {
// TO DO... (definitions)
},
"properties": {
"person": {
"type": "object",
"properties": {
"name": {
"$ref": "#/definitions/PersonType/properties/name"
},
"surname": {
"$ref": "#/definitions/PersonType/properties/surname"
},
"age": {
"$ref": "#/definitions/PersonType/properties/age"
},
"birthDate": {
"type": "string",
"format": "date"
},
"nationality": {
"type": "string",
"minLength": 0,
"maxLength": 30
},
// ... (other Properties for contact, study & work, family)
}
}
}
}
// UI Schema
{
"type": "VerticalLayout",
"elements": [
{
"type": "Group",
"label": "Personal Information",
"elements": [
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/name"
},
{
"type": "Control",
"scope":"#/properties/person/properties/surname"
}
]
},
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/age"
},
{
"type": "Control",
"scope": "#/properties/person/properties/birthDate"
},
{
"type": "Control",
"scope": "#/properties/person/properties/nationality"
}
]
},
// ... (other groups for contact, study & work, family)
]
}
]
}
Step 2: Adding Parent/Guardian Information
Extend the form by adding a section for parent/guardian information. This section will be conditionally shown if the person's age is below 18.
// Schema
{
"title": "A form",
"description": "A simple form example",
"type": "object",
"definitions": {
// TO DO... (definitions)
},
"properties": {
"person": {
"type": "object",
"properties": {
// ... (Previous Properties for name, surname, age, birthdate, and nationality)
"parent": {
"type": "object",
"properties": {
"name": {
"$ref": "#/definitions/PersonType/properties/name"
},
"surname": {
"$ref": "#/definitions/PersonType/properties/surname"
},
"age": {
"$ref": "#/definitions/PersonType/properties/age"
}
}
},
// ... (other Properties for contact, study & work, family)
}
}
}
}
// UI Schema (inside the "Personal Information" group)
{
// ... (previous controls for name, surname, age, birthdate, and nationality)
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/parent/properties/name",
"label": "Parent/Guardian Name"
},
{
"type": "Control",
"scope": "#/properties/person/properties/parent/properties/surname",
"label": "Parent/Guardian Surname"
},
{
"type": "Control",
"scope": "#/properties/person/properties/parent/properties/age",
"label": "Parent/Guardian Age"
}
],
"rule": {
"effect": "SHOW",
"condition": {
"scope": "#/properties/person/properties/age",
"schema": {
"type": "integer",
"minimum": 0,
"maximum": 18
}
}
}
// ... (other groups for contact, study & work, family)
}
}
Step 3: Incorporating Contact Information
Enhance the form by including contact information, including email, phone, and main contact preference.
// Schema
{
"title": "A form",
"description": "A simple form example",
"type": "object",
"definitions": {
// TO DO... (definitions)
},
"properties": {
"person": {
"type": "object",
"properties": {
// ... (Previous Properties for name, surname, age, birthdate, nationality, and parents)
"contact": {
"type": "object",
"properties": {
"email": {
"type": "string",
"format": "email"
},
"phone": {
"type": "string",
"format": "tel"
},
"mainContact": {
"type": "string",
"enum": [
"Email",
"Phone"
]
}
}
},
// ... (other Properties for study & work, family)
}
}
}
}
// UI Schema (inside the "Contact Information" group)
{
// ... (previous controls for name, surname, age, birthdate, nationality, and parents)
{
{
"type": "Group",
"label": "Contact Information",
"elements": [
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/contact/properties/email"
},
{
"type": "Control",
"scope": "#/properties/person/properties/contact/properties/phone"
},
{
"type": "Control",
"scope": "#/properties/person/properties/contact/properties/mainContact",
"options": {
"format": "radio"
}
}
]
}
]
},
// ... (other groups for study & work, family)
}
}
Step 4: Introducing Study & Work Details
Expand the form to gather study and work-related details, such as last grade, study institution, and employment information.
// Schema
{
"title": "A form",
"description": "A simple form example",
"type": "object",
"definitions": {
// TO DO... (definitions)
},
"properties": {
"person": {
"type": "object",
"properties": {
// ... (Previous Properties for name, surname, age, birthdate, nationality, parents, and contact info)
{
"type": "Group",
"label": "Study & Work",
"elements": [
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/lastGrade"
},
{
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/studyInstitution"
},
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/yearGrade"
}
]
}
]
},
{
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/working"
},
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/companyName"
},
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/seniority"
}
],
"rule": {
"effect": "SHOW",
"condition": {
"scope": "#/properties/person/properties/studyWork/properties/working",
"schema": {
"const": true
}
}
}
}
]
}
]
},
// ... (other Properties for family)
}
}
}
}
// UI Schema (inside the "Study & Work" group)
{
// ... (previous controls for name, surname, age, birthdate, nationality, parents, and conctact info)
{
{
{
"type": "Group",
"label": "Study & Work",
"elements": [
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/lastGrade"
},
{
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/studyInstitution"
},
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/yearGrade"
}
]
}
]
},
{
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/working"
},
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/companyName"
},
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/seniority"
}
],
"rule": {
"effect": "SHOW",
"condition": {
"scope": "#/properties/person/properties/studyWork/properties/working",
"schema": {
"const": true
}
}
}
}
]
}
]
},
// ... (other groups for family)
}
}
}
Step 5: Managing Family Information
Take the form to the next level by capturing family-related information, including civil status, spouse/partner details, children's presence, and children's information.
// Schema
{
"title": "A form",
"description": "A simple form example",
"type": "object",
"definitions": {
// TO DO... (definitions)
},
"properties": {
"person": {
"type": "object",
"properties": {
// ... (Previous Properties for name, surname, age, birthdate, nationality, parents, contact info, and study & work)
"civilStatus": {
"type": "string",
"enum": [
"Single",
"Married",
"Divorced",
"Widow",
"Other..."
]
},
"couple": {
"type": "object",
"properties": {
"name": {
"$ref": "#/definitions/PersonType/properties/name"
},
"surname": {
"$ref": "#/definitions/PersonType/properties/surname"
},
"age": {
"$ref": "#/definitions/PersonType/properties/age"
}
}
},
"childrens": {
"type": "boolean"
},
"childs": {
"type": "array",
"items": {
"type": "object",
"required": [
"childName"
],
"properties": {
"name": {
"$ref": "#/definitions/PersonType/properties/name"
},
"surname": {
"$ref": "#/definitions/PersonType/properties/surname"
},
"age": {
"$ref": "#/definitions/PersonType/properties/age"
}
}
}
}
}
}
}
}
// UI Schema (inside the "Family" group)
{
// ... (previous controls for name, surname, age, birthdate, nationality, parents, conctact info, and study & work)
{
"type": "Group",
"label": "Family",
"elements": [
{
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/civilStatus"
},
{
"type": "Label",
"text":" "
},
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/couple/properties/name"
},
{
"type": "Control",
"scope": "#/properties/person/properties/couple/properties/surname"
},
{
"type": "Control",
"scope": "#/properties/person/properties/couple/properties/age"
}
],
"rule": {
"effect": "SHOW",
"condition": {
"scope": "#/properties/person/properties/civilStatus",
"schema": {
"const": "Married"
}
}
}
}
]
},
{
"type": "Control",
"scope": "#/properties/person/properties/childrens"
},
{
"type": "Control",
"scope": "#/properties/person/properties/childs",
"rule": {
"effect": "SHOW",
"condition": {
"scope": "#/properties/person/properties/childrens",
"schema": {
"const": true
}
}
}
}
]
}
}
Congratulations! You've successfully created a dynamic form using KuFlow and JSON Forms. Starting from a simple form, you've gradually built up its complexity to capture a wide range of information. This step-by-step guide showcases the power of KuFlow's form-building capabilities, enabling you to create customized and interactive forms with ease.
Below you can see the form result, and we'll share the entire code
Schema
{
"title": "A form",
"description": "A simple form example",
"type": "object",
"definitions": {
"PersonType": {
"type": "object",
"properties": {
"name": {
"type": "string",
"minLength": 0,
"maxLength": 30
},
"surname": {
"type": "string",
"minLength": 0,
"maxLength": 30
},
"age": {
"type": "integer",
"minimum": 0,
"maximum": 200
}
}
}
},
"properties": {
"person": {
"type": "object",
"properties": {
"name": {
"$ref": "#/definitions/PersonType/properties/name"
},
"surname": {
"$ref": "#/definitions/PersonType/properties/surname"
},
"age": {
"$ref": "#/definitions/PersonType/properties/age"
},
"birthDate": {
"type": "string",
"format": "date"
},
"nationality": {
"type": "string",
"minLength": 0,
"maxLength": 30
},
"parent": {
"type": "object",
"properties": {
"name": {
"$ref": "#/definitions/PersonType/properties/name"
},
"surname": {
"$ref": "#/definitions/PersonType/properties/surname"
},
"age": {
"$ref": "#/definitions/PersonType/properties/age"
}
}
},
"contact": {
"type": "object",
"properties": {
"email": {
"type": "string",
"format": "email"
},
"phone": {
"type": "string",
"format": "tel"
},
"mainContact": {
"type": "string",
"enum": [
"Email",
"Phone"
]
}
}
},
"studyWork": {
"type": "object",
"properties": {
"lastGrade": {
"type": "string",
"enum": [
"Primary School",
"High School",
"Bachelor",
"University"
]
},
"working": {
"type": "boolean"
},
"companyName": {
"type": "string",
"minLength": 0,
"maxLength": 30
},
"seniority": {
"type": "number"
},
"studyInstitution": {
"type": "string",
"minLength": 0,
"maxLength": 30
},
"yearGrade": {
"type": "string",
"pattern": "^(19[5-9][0-9]|20[0-1][0-9]|202[0-3])$"
}
}
},
"civilStatus": {
"type": "string",
"enum": [
"Single",
"Married",
"Divorced",
"Widow",
"Other..."
]
},
"couple": {
"type": "object",
"properties": {
"name": {
"$ref": "#/definitions/PersonType/properties/name"
},
"surname": {
"$ref": "#/definitions/PersonType/properties/surname"
},
"age": {
"$ref": "#/definitions/PersonType/properties/age"
}
}
},
"childrens": {
"type": "boolean"
},
"childs": {
"type": "array",
"items": {
"type": "object",
"required": [
"childName"
],
"properties": {
"name": {
"$ref": "#/definitions/PersonType/properties/name"
},
"surname": {
"$ref": "#/definitions/PersonType/properties/surname"
},
"age": {
"$ref": "#/definitions/PersonType/properties/age"
}
}
}
}
}
}
}
}
UI Schema
{
"type": "VerticalLayout",
"elements": [
{
"type": "Group",
"label": "Personal Information",
"elements": [
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/name"
},
{
"type": "Control",
"scope":"#/properties/person/properties/surname"
}
]
},
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/age"
},
{
"type": "Control",
"scope": "#/properties/person/properties/birthDate"
},
{
"type": "Control",
"scope": "#/properties/person/properties/nationality"
}
]
},
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/parent/properties/name",
"label": "Parent/Guardian Name"
},
{
"type": "Control",
"scope": "#/properties/person/properties/parent/properties/surname",
"label": "Parent/Guardian Surname"
},
{
"type": "Control",
"scope": "#/properties/person/properties/parent/properties/age",
"label": "Parent/Guardian Age"
}
],
"rule": {
"effect": "SHOW",
"condition": {
"scope": "#/properties/person/properties/age",
"schema": {
"type": "integer",
"minimum": 0,
"maximum": 18
}
}
}
}
]
},
{
"type": "Group",
"label": "Contact Information",
"elements": [
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/contact/properties/email"
},
{
"type": "Control",
"scope": "#/properties/person/properties/contact/properties/phone"
},
{
"type": "Control",
"scope": "#/properties/person/properties/contact/properties/mainContact",
"options": {
"format": "radio"
}
}
]
}
]
},
{
"type": "Group",
"label": "Study & Work",
"elements": [
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/lastGrade"
},
{
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/studyInstitution"
},
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/yearGrade"
}
]
}
]
},
{
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/working"
},
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/companyName"
},
{
"type": "Control",
"scope": "#/properties/person/properties/studyWork/properties/seniority"
}
],
"rule": {
"effect": "SHOW",
"condition": {
"scope": "#/properties/person/properties/studyWork/properties/working",
"schema": {
"const": true
}
}
}
}
]
}
]
},
{
"type": "Group",
"label": "Family",
"elements": [
{
"type": "VerticalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/civilStatus"
},
{
"type": "Label",
"text":" "
},
{
"type": "HorizontalLayout",
"elements": [
{
"type": "Control",
"scope": "#/properties/person/properties/couple/properties/name"
},
{
"type": "Control",
"scope": "#/properties/person/properties/couple/properties/surname"
},
{
"type": "Control",
"scope": "#/properties/person/properties/couple/properties/age"
}
],
"rule": {
"effect": "SHOW",
"condition": {
"scope": "#/properties/person/properties/civilStatus",
"schema": {
"const": "Married"
}
}
}
}
]
},
{
"type": "Control",
"scope": "#/properties/person/properties/childrens"
},
{
"type": "Control",
"scope": "#/properties/person/properties/childs",
"rule": {
"effect": "SHOW",
"condition": {
"scope": "#/properties/person/properties/childrens",
"schema": {
"const": true
}
}
}
}
]
}
]
}
Messages
{
"person.studyWork.yearGrade.error.pattern": "Must be between 1950 and 2023"
}
Data (after the form completion)
{
"person": {
"name": "Hector",
"surname": "Tessari",
"age": 38,
"birthDate": "1984-10-25",
"nationality": "Italy",
"couple": {
"name": "Florencia",
"surname": "Bena",
"age": 33
},
"contact": {
"email": "htessar@kuflow.com",
"phone": "+34 645142541",
"mainContact": "Email"
},
"studyWork": {
"lastGrade": "Bachelor",
"studyInstitution": "IFTS5",
"yearGrade": "2017",
"working": true,
"companyName": "KuFlow S.L.",
"seniority": 1
},
"civilStatus": "Married",
"childrens": true,
"childs": [
{
"name": "pepe",
"surname": "pepino",
"age": 1
},
{
"name": "pipa",
"surname": "pipina",
"age": 2
}
]
}
}
For more examples, please visit our Community Examples Section.